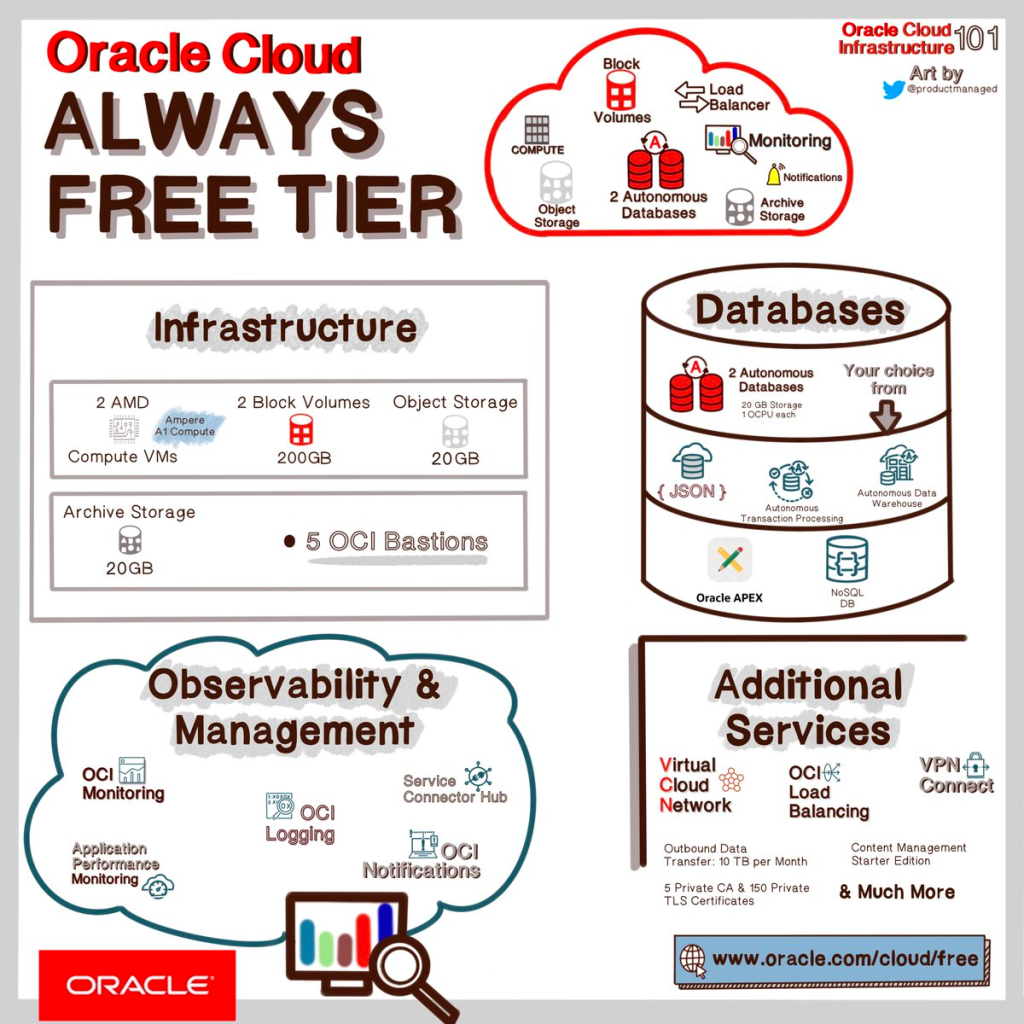
What is Always Free- Oracle OCI.
Posted by Sriram Sanka on October 14, 2023
Posted in Uncategorized | Leave a Comment »
Just WoW !!
Posted by Sriram Sanka on September 20, 2023
Posted in Uncategorized | Leave a Comment »
Which Load Balancer is right for you..?
Posted by Sriram Sanka on September 20, 2023
Oracle has Two Load Balancer Services available. , In case if you are not sure which one is best for you and what are the differences, you can refer the following chart provided by Oracle. https://cloud.oracle.com/load-balancers#
Load balancer
The load balancer service provides a reverse proxy solution that hides the IP of the client from backend application server and vice versa. It is capable of performing advanced layer 7 (HTTP/HTTPS), layer 4 (TCP) load balancing and SSL offloading.
Best for: Websites, mobile apps, SSL termination, and advanced HTTP handling.
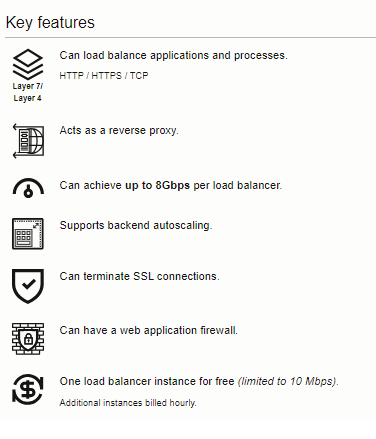
Network load balancer
The network load balancer service provides a pass-through (non-proxy solution) that is capable of preserving the client header (source and destination IP). It is built for speed, optimized for long running connections, high throughput and low latency.
Best for: Scaling network virtual appliances such as firewalls, real-time streaming, long running connections, Voice over IP (VoIP), Internet of Things (IoT), and trading platforms.
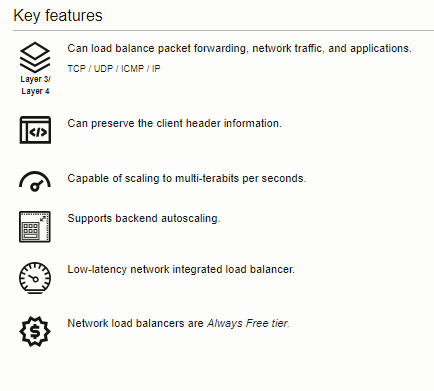
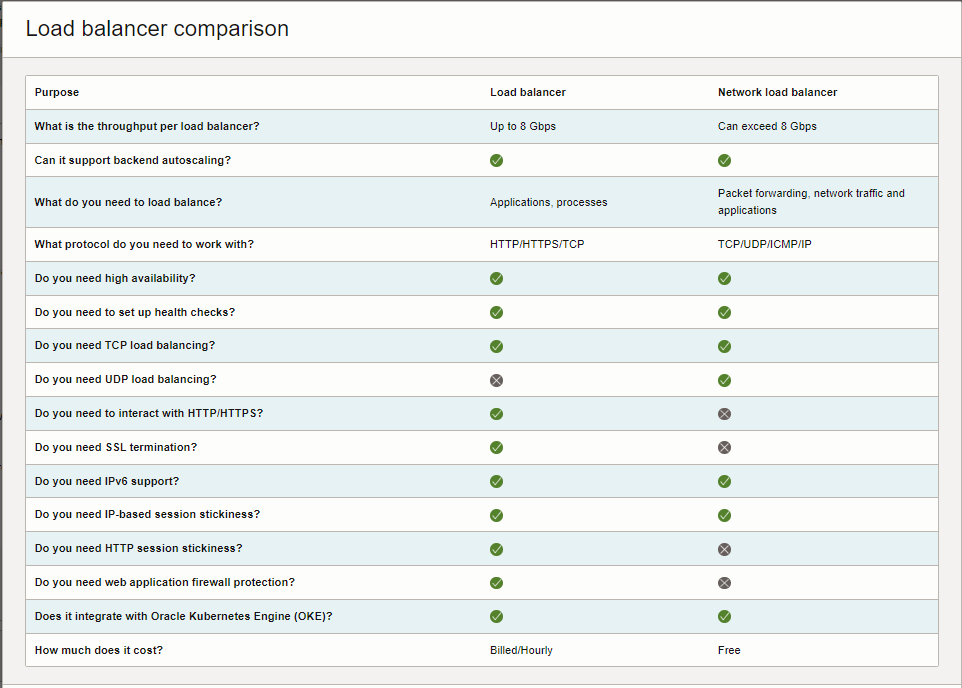
Posted in Cloud, Load Balancer, Network Load Balancer, OCI, oracle, Oracle Cloud Service, Web-Traffic | Tagged: Load Balancer, OCI, Oracle Cloud | Leave a Comment »
Enable/Disable MFA on Oracle Cloud (OCI) portal
Posted by Sriram Sanka on September 12, 2023
When signing up, it is mandatory to configure and enable Multi-Factor Authentication (MFA). Once configured, during the login process, you will be required to obtain approval either through the Oracle Authenticator App or by obtaining a passcode.
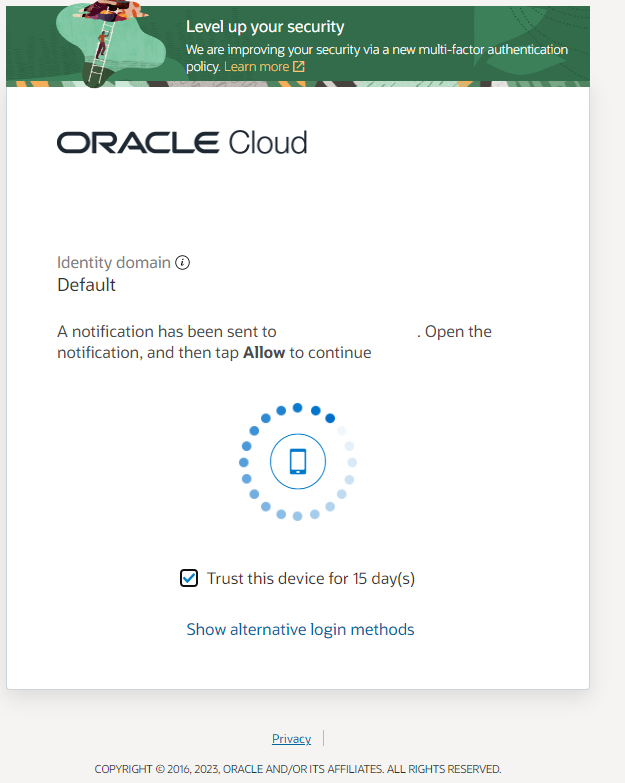
To customise the MFA Configuration, access the Identity & Security section and select the desired domain. This action will direct you to the Domain Dashboard, where you can choose the appropriate security settings and explore additional options for Multi-Factor Authentication.
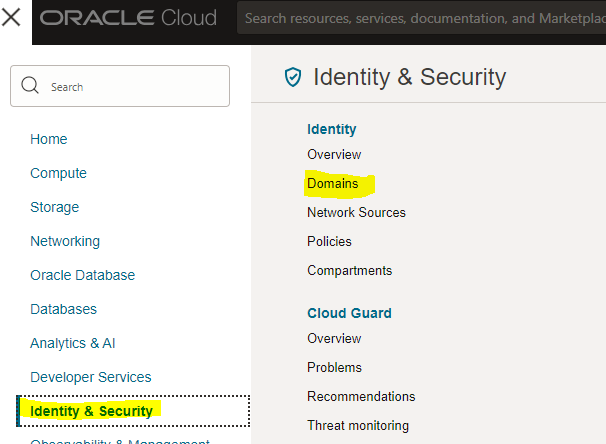
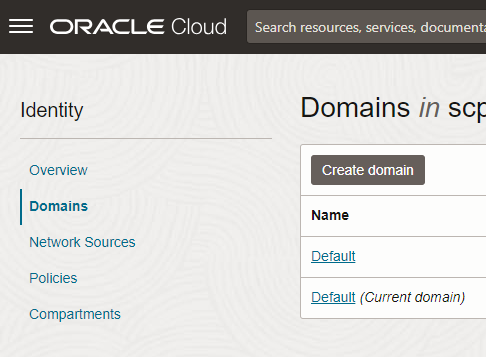
You can change the Default Configuration under Identity–>Domains–>Default domain–>Security –>MFA, when you try to update the changes , you may get the error as below “You cannot disable these factors as they are being referenced in Rules used in Sign-On Policies. Remove them from the Rules before disabling the factors.”
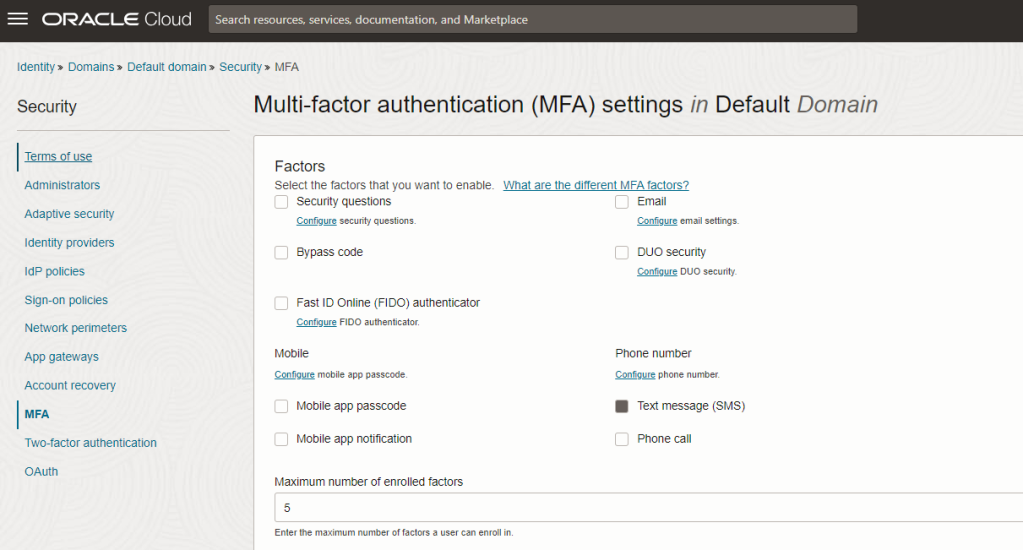
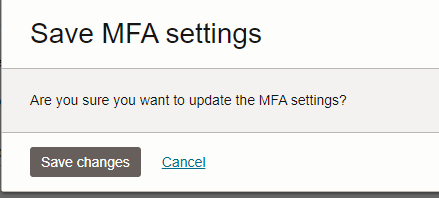

In order to prevent the occurrence of the aforementioned error, it is advisable to disable the default sign-in policy for both users and administrators as indicated in the error message.
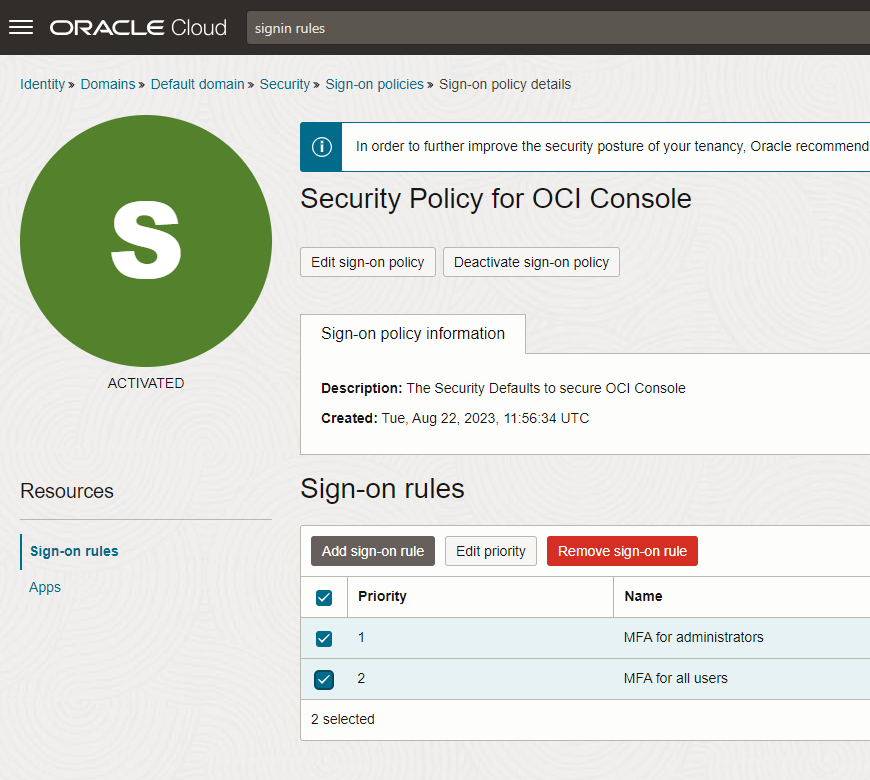
Please note that it is not advisable to disable security policies and multi-factor authentication (MFA) for cloud access. follow https://docs.oracle.com/en-us/iaas/Content/Security/Reference/iam_security_topic-IAM_MFA.htm for more information.
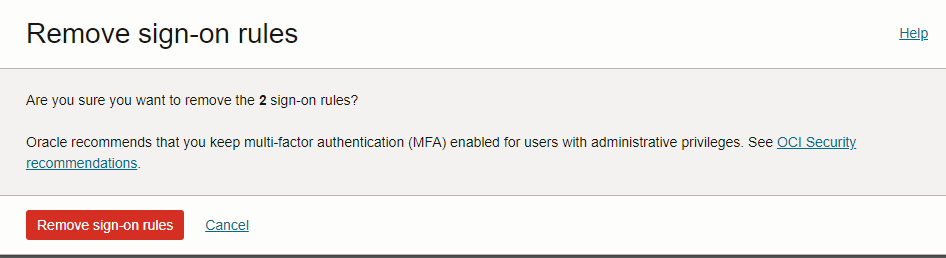
Reference
Posted in MFA, OCI, oracle, Security | Tagged: MFA, OCI, Oracle Cloud, Security | Leave a Comment »
Oracle Offers Free Training and Certification Program as Demand for Cloud and AI Accelerates.
Posted by Sriram Sanka on August 31, 2023
Oracle has extended the Race to Certification Challenge: Free Certifications for OCI until September 30, 2023
Unleash your potential by certifying on Oracle Cloud Infrastructure, free until September 30, 2023:
- Acquire key, in-demand skills in Cloud Computing, Security, AI/ML, DevOps, Autonomous Databases, and more.
- Learn concepts through simple, structured learning paths designed by Oracle expert instructors and by leveraging our learning community.
- Choose from 21 core certification courses covering Oracle Cloud Infrastructure.
How do I get started?
- Visit OCI Certification Promotion page and select your certification learning path.
- Sign in to Oracle MyLearn using an Oracle SSO account. If you don’t have an Oracle SSO account, create one when you access one of the learning paths below.
- Join the Race to Certification Challenge.
- Prepare for your certification exam by leveraging the learning path content, attending a live instructor-led event, or asking a question in our learning community
- Share your certification success on social Media: #OracleCertified
Certification Learning Paths
- OCI 2023 Foundations
- OCI 2023 Architect Associate
- OCI 2023 Multicloud Architect Associate
- OCI 2023 Security Professional
- OCI 2023 Developer Professional
- OCI 2023 DevOps Professional
- OCI 2023 Cloud Operations Professional
- OCI 2023 Data Science Professional
- OCI 2023 Observability Professional
- OCI 2023 Application Integration Professional
- OCI 2023 Digital Assistant Professional
- Oracle Cloud Data Management 2023 Foundations
- Oracle Autonomous Database Cloud 2023 Professional
- Oracle Cloud Database 2023 Migration and Integration Professional
- Oracle Base Database Services 2023 Professional
- Oracle Machine Learning using Autonomous Database 2023 Associate
For more details, visit Free Certification for OCI.
Posted in Uncategorized | Leave a Comment »
Download Youtube ( Channel ) videos using Python Module – Pytube ,scrapetube.
Posted by Sriram Sanka on April 25, 2023
In this post, I will present the code to download Youtube Individual/Channel Videos step-by-step.
You can download the Videos using pytube module by passing the video URL as an argument. Just Install pytube and run as below.
python -m pip install pytube

This will download the given video URL to your current Directory, what about if you want to store your local copy for your educational purpose when you are offline.? The following will download the all the videos from the given URL to your desired location in the PC.
import requests
import re
from bs4 import BeautifulSoup
from pytube.cli import on_progress
from pytube import YouTube
from pytube import Playlist
from pytube import Channel
def get_youtube(i):
try:
yt = YouTube(i,on_progress_callback=on_progress)
yt.streams.filter(progressive=True, file_extension='mp4').order_by('resolution').desc().first().download('C:\\Users\\Dell\\Videos\\downs\\Sriram_Channel\\')
except:
print(f'\nError in downloading: {yt.title} from -->' + i)
pass
l = Channel('https://www.youtube.com/channel/UCw43xCtkl26vGIGtzBoaWEw')
for video in l.video_urls:
get_youtube(video)
You may get regular Expression error in fetching the video information due to changes implemented in the channel URL using symbols. or any other restrictions which are unsupported by the pytube module, either you have to download and install the latest or identify and fix it on your own.
To avoid this, you can use another module called scrapetube
pip install scrapetube

The following code will give you the Video id from the URL provided as input. you need to append ‘https://www.youtube.com/watch?v=’ to mark that as a complete URL.
import scrapetube
videos = scrapetube.get_channel("........t7XvGJ3AGpSon.......")
for video in videos:
print('https://www.youtube.com/watch?v='+ video['videoId'])
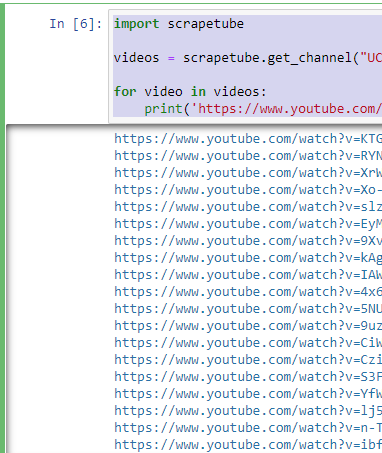
as you can see channel id is the key here, what if you are unaware of channel id.? You can get the channel id either from browser view page source or you can also use “requests” and “BeautifulSoup” module to get the channel ID
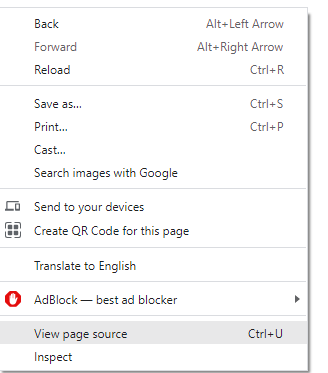

import requests
from bs4 import BeautifulSoup
url = input("Please Enter youtube channel URL ")
response = requests.get(url)
soup = BeautifulSoup(response.content,'html.parser')
print(soup.find("meta", itemprop="channelId")['content'])
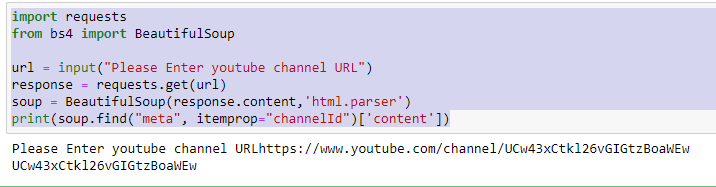
Instead of using Channel from pytube module, to make it easier lets make changes to the original code snippet posted above, using module “scrapetube.get_channel “
import requests
import re
from bs4 import BeautifulSoup
from pytube.cli import on_progress
from pytube import YouTube
from pytube import Playlist
from pytube import Channel
import scrapetube
import json
def get_youtube(i):
try:
print('Downloading ' +i)
yt = YouTube(i,on_progress_callback=on_progress)
#print(f'\nStarted downloading: {yt} from -->' + i)
yt.streams.filter(progressive=True, file_extension='mp4').order_by('resolution').desc().first().download('C:\\Users\\Dell\\Videos\\downs\\Sriram_Channel\\')
except:
print('Error Downloading' +i)
pass
l = scrapetube.get_channel("UCw43xCtkl26vGIGtzBoaWEw")
for video in l:
#print('Downloading https://www.youtube.com/watch?v='+ video['videoId'])
get_youtube('https://www.youtube.com/watch?v='+ video['videoId'])
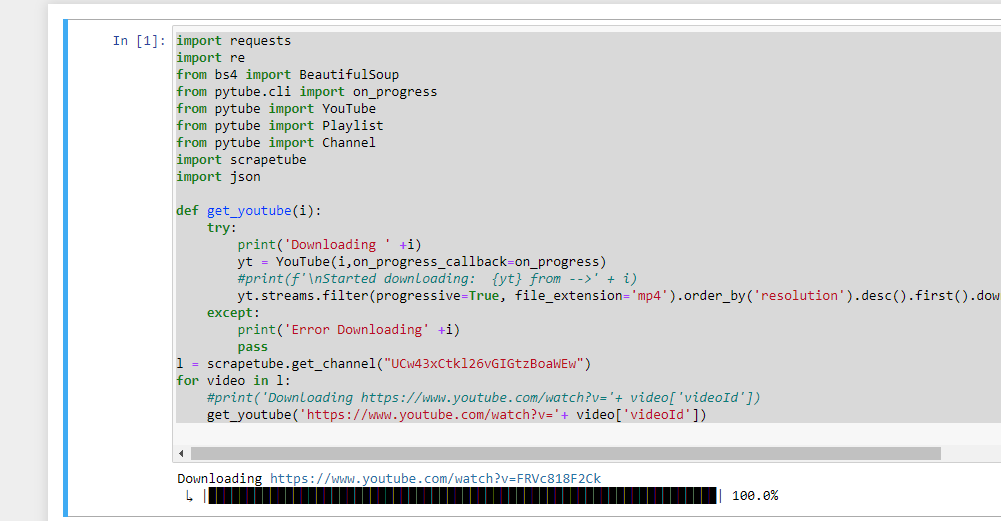
Hope you like it ! Happy Reading.
Posted in Python, pytube, scrapetube, WebScraping, youtube | Tagged: DOWNLOAD, Python, pytube, scrapetube, web-scraping, youtube, youtube channel | Leave a Comment »
Reading Chrome Bookmarks using Python Module chrome_bookmarks
Posted by Sriram Sanka on April 24, 2023
Chrome Browser Bookmark file is in JSON format whereas history file is a database format. One can read the data file using SQLite(DB Browser) to get the URL and downloads data.
In this post I am using chrome-bookmarks module to read the bookmarks and print the URL along with it Name and Folder Information.
Install chrome-bookmarks module using pip
pip install chrome-bookmarks

Now you can read the URL from Python as below.
import chrome_bookmarks
for url in chrome_bookmarks.urls:
print(url.url)

We Can also print the URL Description/Name as below.
import chrome_bookmarks
for url in chrome_bookmarks.urls:
print(url.url, url.name)
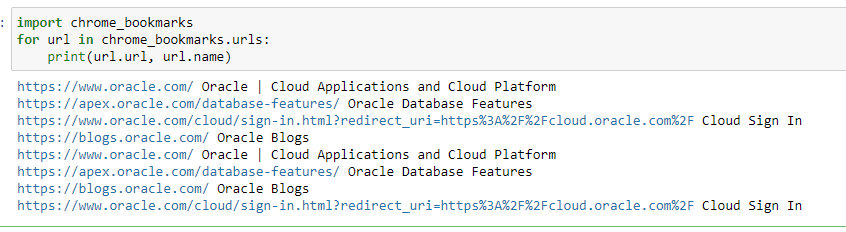
import chrome_bookmarks
for folder in chrome_bookmarks.folders:
print(folder.name)
print(folder.folders)
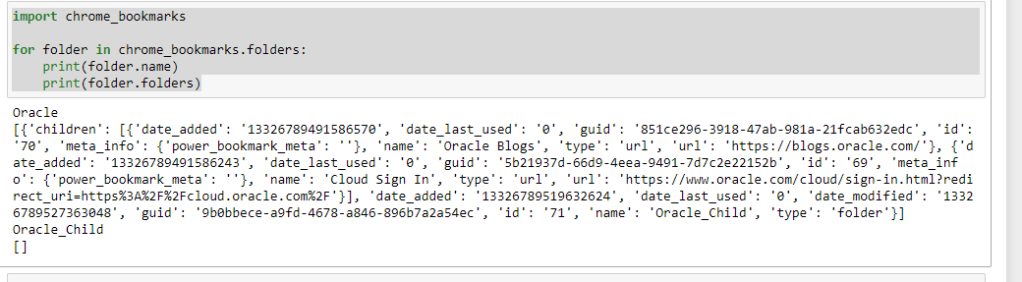
It is also possible to read the JSON without using the above module.
import json
file ="C:\\Users\\Dell\\AppData\\Local\\Google\\Chrome\\User Data\\Default\\Bookmarks"
with open(file, "r", encoding='utf-8') as bookmarks:
bookmark_data = json.load(bookmarks)
print(json.dumps(bookmark_data, indent=1))
{
"checksum": "7b7d115080ddda0dcab6b428f64aa3a5",
"roots": {
"bookmark_bar": {
"children": [
{
"date_added": "13326789370504643",
"date_last_used": "0",
"guid": "5c3e2329-1d0c-4a9d-b85e-426d6fd31301",
"id": "62",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle | Cloud Applications and Cloud Platform",
"type": "url",
"url": "https://www.oracle.com/"
},
{
"date_added": "13326789428536219",
"date_last_used": "0",
"guid": "0a972887-7f34-41b3-bf59-1f2f2f521a0e",
"id": "63",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle Database Features",
"type": "url",
"url": "https://apex.oracle.com/database-features/"
},
{
"date_added": "13326789439984046",
"date_last_used": "0",
"guid": "25e1d231-a825-46fd-a8a9-829bf07dc5bf",
"id": "64",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Cloud Sign In",
"type": "url",
"url": "https://www.oracle.com/cloud/sign-in.html?redirect_uri=https%3A%2F%2Fcloud.oracle.com%2F"
},
{
"date_added": "13326789456087992",
"date_last_used": "0",
"guid": "a0e39859-9596-47be-bc61-d6099f152fb1",
"id": "65",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle Blogs",
"type": "url",
"url": "https://blogs.oracle.com/"
},
{
"children": [
{
"date_added": "13326789491584887",
"date_last_used": "0",
"guid": "b6f90dec-e303-4a2b-bb53-a7357c8d694f",
"id": "67",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle | Cloud Applications and Cloud Platform",
"type": "url",
"url": "https://www.oracle.com/"
},
{
"date_added": "13326789491585797",
"date_last_used": "0",
"guid": "ffeea96a-87d7-4053-b674-7ac9cfb50a13",
"id": "68",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle Database Features",
"type": "url",
"url": "https://apex.oracle.com/database-features/"
},
{
"children": [
{
"date_added": "13326789491586570",
"date_last_used": "0",
"guid": "851ce296-3918-47ab-981a-21fcab632edc",
"id": "70",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Oracle Blogs",
"type": "url",
"url": "https://blogs.oracle.com/"
},
{
"date_added": "13326789491586243",
"date_last_used": "0",
"guid": "5b21937d-66d9-4eea-9491-7d7c2e22152b",
"id": "69",
"meta_info": {
"power_bookmark_meta": ""
},
"name": "Cloud Sign In",
"type": "url",
"url": "https://www.oracle.com/cloud/sign-in.html?redirect_uri=https%3A%2F%2Fcloud.oracle.com%2F"
}
],
"date_added": "13326789519632624",
"date_last_used": "0",
"date_modified": "13326789527363048",
"guid": "9b0bbece-a9fd-4678-a846-896b7a2a54ec",
"id": "71",
"name": "Oracle_Child",
"type": "folder"
}
],
"date_added": "13326789485096843",
"date_last_used": "0",
"date_modified": "13326789519632800",
"guid": "ea6663fa-05df-4751-b25b-125561aed88d",
"id": "66",
"name": "Oracle",
"type": "folder"
}
],
"date_added": "13326774450786953",
"date_last_used": "0",
"date_modified": "13326789491586570",
"guid": "0bc5d13f-2cba-5d74-951f-3f233fe6c908",
"id": "1",
"name": "Bookmarks bar",
"type": "folder"
},
"other": {
"children": [],
"date_added": "13326774450786955",
"date_last_used": "0",
"date_modified": "0",
"guid": "82b081ec-3dd3-529c-8475-ab6c344590dd",
"id": "2",
"name": "Other bookmarks",
"type": "folder"
},
"synced": {
"children": [],
"date_added": "13326774450786957",
"date_last_used": "0",
"date_modified": "0",
"guid": "4cf2e351-0e85-532b-bb37-df045d8f8d0f",
"id": "3",
"name": "Mobile bookmarks",
"type": "folder"
}
},
"version": 1
}
Hope you like it. ! Happy learning.
Posted in Python | Tagged: Python | Leave a Comment »
Step By Step Instructions on Installing Azure ARM template test toolkit
Posted by Sriram Sanka on April 5, 2023
Azure ARM Template Validation Tool Kit helps you to validate & meet the Requirements to Publish your Solution to Azure Market Place.
You can Download the Latest Toolkit from https://github.com/Azure/arm-ttk/releases .
- Download and Extract(unzip) arm-ttk.zip file
- Start the Power Shell as administrator
- Navigate to the Extracted Folder/Directory.
- Unblock the script files Using “Get-ChildItem *.ps1, *.psd1, *.ps1xml, *.psm1 -Recurse | Unblock-File”
- Import the module using ” Import-Module .\arm-ttk.psd1 “
- Validate Templates using module “Test-AzTemplate”
Get-ChildItem *.ps1, *.psd1, *.ps1xml, *.psm1 -Recurse | Unblock-File
Import-Module .\arm-ttk.psd1
Test-AzTemplate -TemplatePath \path\to\template
Download and Extract(unzip) arm-ttk.zip file

Start the Power Shell as administrator & Navigate to the Extracted Folder/Directory.
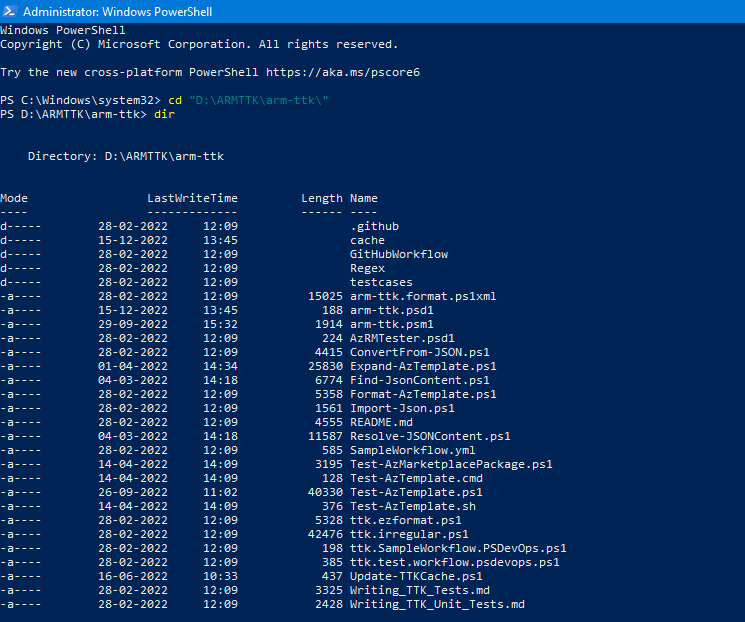
Unblock the script files Using “Get-ChildItem *.ps1, *.psd1, *.ps1xml, *.psm1 -Recurse | Unblock-File”

Import the module using ” Import-Module .\arm-ttk.psd1 “

As mentioned in the Error, we need to Enable Execution Policy as Remote Signed to Local Machine to avoid the above Error.
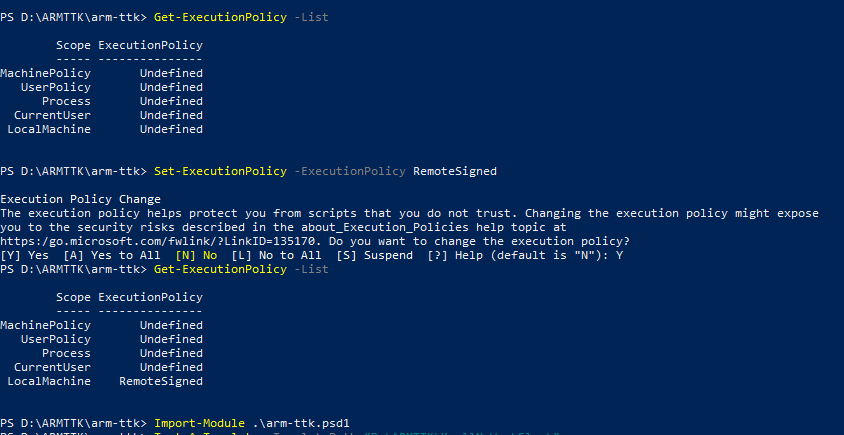
Note : If you do not wish to Import the Module, You can still run the Test-AzTemplate as Local using “./Test-AzTemplate ” check the help provided for more Details.
Run “Test-AzTemplate -TemplatePath \path\to\template” to validate your ARM Templates (In My Case UI Definition and MainTemplate)
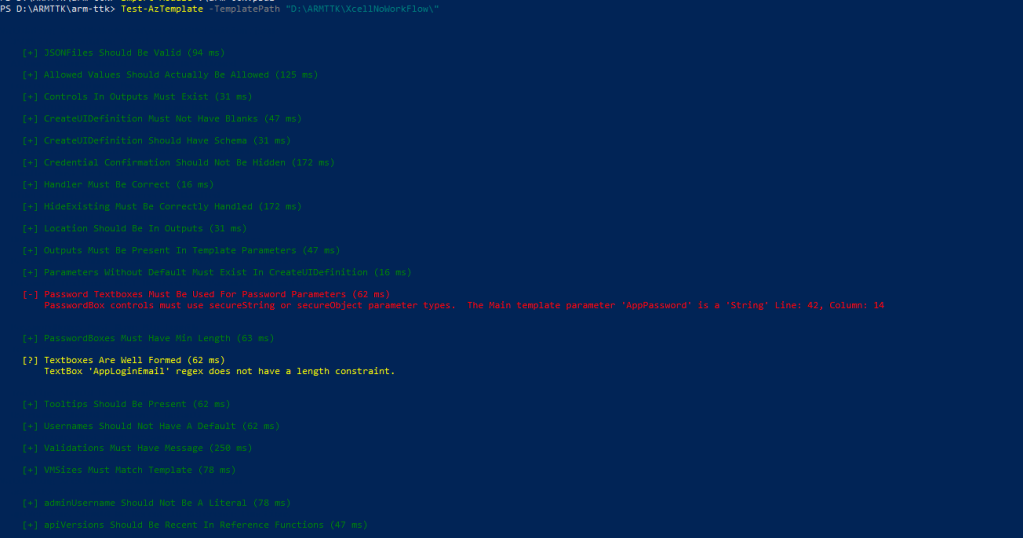
Fix the Reported issues and revalidate.
PS D:\ARMTTK\arm-ttk> Test-AzTemplate -TemplatePath "D:\ARMTTK\XcellNoWorkFlow\" Validating XcellNoWorkFlow\createUiDefinition.json JSONFiles Should Be Valid [+] JSONFiles Should Be Valid (30 ms) Allowed Values Should Actually Be Allowed [+] Allowed Values Should Actually Be Allowed (100 ms) Controls In Outputs Must Exist [+] Controls In Outputs Must Exist (19 ms) CreateUIDefinition Must Not Have Blanks [+] CreateUIDefinition Must Not Have Blanks (21 ms) CreateUIDefinition Should Have Schema [+] CreateUIDefinition Should Have Schema (13 ms) Credential Confirmation Should Not Be Hidden [+] Credential Confirmation Should Not Be Hidden (93 ms) Handler Must Be Correct [+] Handler Must Be Correct (10 ms) HideExisting Must Be Correctly Handled [+] HideExisting Must Be Correctly Handled (154 ms) Location Should Be In Outputs [+] Location Should Be In Outputs (9 ms) Outputs Must Be Present In Template Parameters [+] Outputs Must Be Present In Template Parameters (10 ms) Parameters Without Default Must Exist In CreateUIDefinition [+] Parameters Without Default Must Exist In CreateUIDefinition (20 ms) Password Textboxes Must Be Used For Password Parameters [+] Password Textboxes Must Be Used For Password Parameters (65 ms) PasswordBoxes Must Have Min Length [+] PasswordBoxes Must Have Min Length (60 ms) Textboxes Are Well Formed [?] Textboxes Are Well Formed (66 ms) TextBox 'AppLoginEmail' regex does not have a length constraint. Tooltips Should Be Present [+] Tooltips Should Be Present (66 ms) Usernames Should Not Have A Default [+] Usernames Should Not Have A Default (61 ms) Validations Must Have Message [+] Validations Must Have Message (60 ms) VMSizes Must Match Template [+] VMSizes Must Match Template (62 ms) Validating XcellNoWorkFlow\mainTemplate.json adminUsername Should Not Be A Literal [+] adminUsername Should Not Be A Literal (59 ms) apiVersions Should Be Recent In Reference Functions [+] apiVersions Should Be Recent In Reference Functions (11 ms) apiVersions Should Be Recent [+] apiVersions Should Be Recent (117 ms)
artifacts parameter
[+] artifacts parameter (18 ms)
CommandToExecute Must Use ProtectedSettings For Secrets
[+] CommandToExecute Must Use ProtectedSettings For Secrets (68 ms)
DependsOn Best Practices
[+] DependsOn Best Practices (59 ms)
Deployment Resources Must Not Be Debug
[+] Deployment Resources Must Not Be Debug (56 ms)
DeploymentTemplate Must Not Contain Hardcoded Uri
[+] DeploymentTemplate Must Not Contain Hardcoded Uri (14 ms)
DeploymentTemplate Schema Is Correct
[+] DeploymentTemplate Schema Is Correct (9 ms)
Dynamic Variable References Should Not Use Concat
[+] Dynamic Variable References Should Not Use Concat (12 ms)
IDs Should Be Derived From ResourceIDs
[+] IDs Should Be Derived From ResourceIDs (67 ms)
Location Should Not Be Hardcoded
[+] Location Should Not Be Hardcoded (154 ms)
ManagedIdentityExtension must not be used
[+] ManagedIdentityExtension must not be used (10 ms)
Min And Max Value Are Numbers
[+] Min And Max Value Are Numbers (10 ms)
Outputs Must Not Contain Secrets
[+] Outputs Must Not Contain Secrets (12 ms)
Parameter Types Should Be Consistent
[+] Parameter Types Should Be Consistent (87 ms)
Parameters Must Be Referenced
[+] Parameters Must Be Referenced (52 ms)
Password params must be secure
[+] Password params must be secure (16 ms)
providers apiVersions Is Not Permitted
[+] providers apiVersions Is Not Permitted (18 ms)
ResourceIds should not contain
[+] ResourceIds should not contain (22 ms)
Resources Should Have Location
[+] Resources Should Have Location (11 ms)
Resources Should Not Be Ambiguous
[+] Resources Should Not Be Ambiguous (12 ms)
Secure Params In Nested Deployments
[+] Secure Params In Nested Deployments (56 ms)
Secure String Parameters Cannot Have Default
[+] Secure String Parameters Cannot Have Default (12 ms)
Template Should Not Contain Blanks
[+] Template Should Not Contain Blanks (164 ms)
URIs Should Be Properly Constructed
[+] URIs Should Be Properly Constructed (173 ms)
Variables Must Be Referenced
[+] Variables Must Be Referenced (11 ms)
Virtual Machines Should Not Be Preview
[+] Virtual Machines Should Not Be Preview (64 ms)
VM Images Should Use Latest Version
[+] VM Images Should Use Latest Version (9 ms)
VM Size Should Be A Parameter
[+] VM Size Should Be A Parameter (82 ms)
Pass : 48
Fail : 0
Total : 48
Restrict the Execution Policy to its previous state.
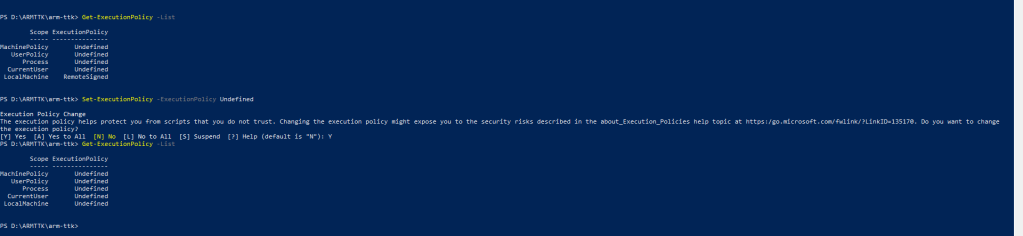
Hope you find it useful, Happy Learning.
Posted in ARM Template, ARM-TTK, Azure, Cloud, Installation, Microsoft Azure Cloud ARM Template | Tagged: ARM, Azure, Cloud, Installaiton, powershell, TEMPLATE, TTK | Leave a Comment »
Oracle Database 21c Express Edition (XE) RPM Installation On Oracle Linux 9
Posted by Sriram Sanka on March 29, 2023
What is Oracle Express Edition
Oracle Database Express Edition (XE) is a community supported edition of the Oracle Database family. It can be Installed in Linux, Windows, Dockers, and Virtual Machine as well.
Oracle Database Express Edition does not restrict in which environment it can be deployed. However, Oracle Database Express Edition is not supported and does not receive any patches, including security patches.
Oracle Database XE supports up to:
- 2 CPUs for foreground processes
- 2GB of RAM (SGA and PGA combined)
- 12GB of user data on disk (irrespective of compression factor)
You can Download Oracle XE from https://www.oracle.com/nl/database/technologies/xe-downloads.html but its not available for Oracle Linux Version 9, In this article we are going install OL8 Version in OL9.
Pre- Installation
1. Make sure to have FQDN(fully qualified domain name) and an Entry with the same in /etc/hosts file.
2. Install dependent rpm compat-openssl10 , This can be downloaded from https://dl.rockylinux.org/pub/rocky/8/AppStream/x86_64/os/Packages/c/compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm
wget https://dl.rockylinux.org/pub/rocky/8/AppStream/x86_64/os/Packages/c/compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm
--2022-12-22 07:06:53-- https://dl.rockylinux.org/pub/rocky/8/AppStream/x86_64/os/Packages/c/compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm
Resolving dl.rockylinux.org (dl.rockylinux.org)... 199.232.194.132, 199.232.198.132, 2a04:4e42:4d::644, ...
Connecting to dl.rockylinux.org (dl.rockylinux.org)|199.232.194.132|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1182640 (1.1M) [application/x-redhat-package-manager]
Saving to: ‘compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm’
compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm 100%[========================================================================================================================================>] 1.13M --.-KB/s in 0.04s
2022-12-22 07:06:53 (30.2 MB/s) - ‘compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm’ saved [1182640/1182640]
dnf -y localinstall compat-openssl10-1.0.2o-4.el8_6.x86_64.rpm
Last metadata expiration check: 3:41:22 ago on Thu 22 Dec 2022 03:25:41 AM EST.
Dependencies resolved.
=============================================================================================================================================================================================================================================
Package Architecture Version Repository Size
=============================================================================================================================================================================================================================================
Installing:
compat-openssl10 x86_64 1:1.0.2o-4.el8_6 @commandline 1.1 M
Installing dependencies:
make x86_64 1:4.3-7.el9 ol9_baseos_latest 571 k
Transaction Summary
=============================================================================================================================================================================================================================================
Install 2 Packages
Total size: 1.7 M
Total download size: 571 k
Installed size: 4.5 M
Downloading Packages:
make-4.3-7.el9.x86_64.rpm 267 kB/s | 571 kB 00:02
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Total 267 kB/s | 571 kB 00:02
Running transaction check
Transaction check succeeded.
Running transaction test
Transaction test succeeded.
Running transaction
Preparing : 1/1
Installing : make-1:4.3-7.el9.x86_64 1/2
Installing : compat-openssl10-1:1.0.2o-4.el8_6.x86_64 2/2
Running scriptlet: compat-openssl10-1:1.0.2o-4.el8_6.x86_64 2/2
Verifying : make-1:4.3-7.el9.x86_64 1/2
Verifying : compat-openssl10-1:1.0.2o-4.el8_6.x86_64 2/2
Installed:
compat-openssl10-1:1.0.2o-4.el8_6.x86_64 make-1:4.3-7.el9.x86_64
Complete!
Download and Install Required Softwares
Download , Install the PreInstall RPM and Database RPM using dnf / yum .
# OL8
#dnf install -y oracle-database-preinstall-21c
curl -o oracle-database-preinstall-21c-1.0-1.el8.x86_64.rpm https://yum.oracle.com/repo/OracleLinux/OL8/appstream/x86_64/getPackage/oracle-database-preinstall-21c-1.0-1.el8.x86_64.rpm
dnf -y localinstall oracle-database-xe-21c-1.0-1.ol8.x86_64.rpm
Downloading Database Software RPM
curl -o oracle-database-preinstall-21c-1.0-1.el8.x86_64.rpm https://yum.oracle.com/repo/OracleLinux/OL8/appstream/x86_64/getPackage/oracle-database-preinstall-21c-1.0-1.el8.x86_64.rpm
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 30772 100 30772 0 0 132k 0 --:--:-- --:--:-- --:--:-- 132k
Install PreInstall RPM
yum -y localinstall oracle-database-preinstall-21c-1.0-1.el8.x86_64.rpm
Last metadata expiration check: 3:41:31 ago on Thu 22 Dec 2022 03:25:41 AM EST.
Dependencies resolved.
=============================================================================================================================================================================================================================================
Package Architecture Version Repository Size
=============================================================================================================================================================================================================================================
Installing:
oracle-database-preinstall-21c x86_64 1.0-1.el8 @commandline 30 k
Installing dependencies:
bc x86_64 1.07.1-14.el9 ol9_baseos_latest 135 k
bind-libs x86_64 32:9.16.23-5.el9_1 ol9_appstream 1.2 M
bind-license noarch 32:9.16.23-5.el9_1 ol9_appstream 13 k
bind-utils x86_64 32:9.16.23-5.el9_1 ol9_appstream 224 k
binutils x86_64 2.35.2-24.0.1.el9 ol9_baseos_latest 4.8 M
binutils-gold x86_64 2.35.2-24.0.1.el9 ol9_baseos_latest 743 k
checkpolicy x86_64 3.4-1.el9 ol9_appstream 355 k
fstrm x86_64 0.6.1-3.el9 ol9_appstream 28 k
glibc-devel x86_64 2.34-40.0.1.el9 ol9_appstream 62 k
glibc-headers x86_64 2.34-40.0.1.el9 ol9_appstream 910 k
gssproxy x86_64 0.8.4-4.el9 ol9_baseos_latest 120 k
initscripts x86_64 10.11.5-1.el9 ol9_baseos_latest 287 k
kernel-headers x86_64 5.14.0-162.6.1.el9_1 ol9_appstream 4.2 M
keyutils x86_64 1.6.1-4.el9 ol9_baseos_latest 74 k
ksh x86_64 3:1.0.0~beta.1-2.0.1.el9 ol9_appstream 891 k
libICE x86_64 1.0.10-8.el9 ol9_appstream 71 k
libSM x86_64 1.2.3-10.el9 ol9_appstream 42 k
libX11 x86_64 1.7.0-7.el9 ol9_appstream 648 k
libX11-common noarch 1.7.0-7.el9 ol9_appstream 350 k
libX11-xcb x86_64 1.7.0-7.el9 ol9_appstream 12 k
libXau x86_64 1.0.9-8.el9 ol9_appstream 36 k
libXcomposite x86_64 0.4.5-7.el9 ol9_appstream 29 k
libXext x86_64 1.3.4-8.el9 ol9_appstream 40 k
libXi x86_64 1.7.10-8.el9 ol9_appstream 40 k
libXinerama x86_64 1.1.4-10.el9 ol9_appstream 15 k
libXmu x86_64 1.1.3-8.el9 ol9_appstream 79 k
libXrandr x86_64 1.5.2-8.el9 ol9_appstream 28 k
libXrender x86_64 0.9.10-16.el9 ol9_appstream 28 k
libXt x86_64 1.2.0-6.el9 ol9_appstream 180 k
libXtst x86_64 1.2.3-16.el9 ol9_appstream 21 k
libXv x86_64 1.0.11-16.el9 ol9_appstream 19 k
libXxf86dga x86_64 1.1.5-8.el9 ol9_appstream 21 k
libXxf86vm x86_64 1.1.4-18.el9 ol9_appstream 19 k
libdmx x86_64 1.1.4-12.el9 ol9_appstream 17 k
libev x86_64 4.33-5.el9 ol9_baseos_latest 53 k
libmaxminddb x86_64 1.5.2-3.el9 ol9_appstream 34 k
libnfsidmap x86_64 1:2.5.4-15.el9 ol9_baseos_latest 72 k
libnsl x86_64 2.34-40.0.1.el9 ol9_baseos_latest 69 k
libuv x86_64 1:1.42.0-1.el9 ol9_appstream 158 k
libverto-libev x86_64 0.3.2-3.el9 ol9_baseos_latest 14 k
libxcb x86_64 1.13.1-9.el9 ol9_appstream 251 k
libxcrypt-devel x86_64 4.4.18-3.el9 ol9_appstream 42 k
lm_sensors-libs x86_64 3.6.0-10.el9 ol9_appstream 42 k
net-tools x86_64 2.0-0.62.20160912git.el9 ol9_baseos_latest 344 k
nfs-utils x86_64 1:2.5.4-15.el9 ol9_baseos_latest 507 k
pcp-conf x86_64 5.3.7-7.el9 ol9_appstream 38 k
pcp-libs x86_64 5.3.7-7.el9 ol9_appstream 612 k
policycoreutils-python-utils noarch 3.4-4.el9 ol9_appstream 111 k
protobuf-c x86_64 1.3.3-12.el9 ol9_baseos_latest 40 k
python3-audit x86_64 3.0.7-103.el9 ol9_appstream 88 k
python3-libsemanage x86_64 3.4-2.el9 ol9_appstream 85 k
python3-policycoreutils noarch 3.4-4.el9 ol9_appstream 2.3 M
python3-pyyaml x86_64 5.4.1-6.0.1.el9 ol9_baseos_latest 258 k
python3-setools x86_64 4.4.0-5.el9 ol9_baseos_latest 795 k
python3-setuptools noarch 53.0.0-10.el9 ol9_baseos_latest 1.3 M
quota x86_64 1:4.06-6.el9 ol9_baseos_latest 212 k
quota-nls noarch 1:4.06-6.el9 ol9_baseos_latest 84 k
rpcbind x86_64 1.2.6-5.el9 ol9_baseos_latest 69 k
smartmontools x86_64 1:7.2-6.el9 ol9_baseos_latest 578 k
sssd-nfs-idmap x86_64 2.7.3-4.0.1.el9_1.1 ol9_baseos_latest 45 k
sysstat x86_64 12.5.4-3.0.1.el9 ol9_appstream 539 k
unzip x86_64 6.0-56.0.1.el9 ol9_baseos_latest 195 k
xorg-x11-utils x86_64 7.5-40.el9 ol9_appstream 123 k
xorg-x11-xauth x86_64 1:1.1-10.el9 ol9_appstream 36 k
Transaction Summary
=============================================================================================================================================================================================================================================
Install 65 Packages
Total size: 25 M
Total download size: 25 M
Installed size: 73 M
Downloading Packages:
(1/64): bc-1.07.1-14.el9.x86_64.rpm 58 kB/s | 135 kB 00:02
(2/64): gssproxy-0.8.4-4.el9.x86_64.rpm 2.8 MB/s | 120 kB 00:00
(3/64): binutils-gold-2.35.2-24.0.1.el9.x86_64.rpm 286 kB/s | 743 kB 00:02
(4/64): keyutils-1.6.1-4.el9.x86_64.rpm 2.6 MB/s | 74 kB 00:00
(5/64): libev-4.33-5.el9.x86_64.rpm 2.2 MB/s | 53 kB 00:00
(6/64): libnfsidmap-2.5.4-15.el9.x86_64.rpm 2.5 MB/s | 72 kB 00:00
(7/64): binutils-2.35.2-24.0.1.el9.x86_64.rpm 1.7 MB/s | 4.8 MB 00:02
(8/64): libverto-libev-0.3.2-3.el9.x86_64.rpm 208 kB/s | 14 kB 00:00
(9/64): net-tools-2.0-0.62.20160912git.el9.x86_64.rpm 8.0 MB/s | 344 kB 00:00
(10/64): nfs-utils-2.5.4-15.el9.x86_64.rpm 8.6 MB/s | 507 kB 00:00
(11/64): protobuf-c-1.3.3-12.el9.x86_64.rpm 39 kB/s | 40 kB 00:01
(12/64): python3-pyyaml-5.4.1-6.0.1.el9.x86_64.rpm 5.1 MB/s | 258 kB 00:00
(13/64): libnsl-2.34-40.0.1.el9.x86_64.rpm 49 kB/s | 69 kB 00:01
(14/64): python3-setools-4.4.0-5.el9.x86_64.rpm 11 MB/s | 795 kB 00:00
(15/64): initscripts-10.11.5-1.el9.x86_64.rpm 122 kB/s | 287 kB 00:02
(16/64): quota-4.06-6.el9.x86_64.rpm 153 kB/s | 212 kB 00:01
(17/64): quota-nls-4.06-6.el9.noarch.rpm 104 kB/s | 84 kB 00:00
(18/64): rpcbind-1.2.6-5.el9.x86_64.rpm 1.8 MB/s | 69 kB 00:00
(19/64): sssd-nfs-idmap-2.7.3-4.0.1.el9_1.1.x86_64.rpm 1.4 MB/s | 45 kB 00:00
(20/64): unzip-6.0-56.0.1.el9.x86_64.rpm 3.4 MB/s | 195 kB 00:00
(21/64): bind-libs-9.16.23-5.el9_1.x86_64.rpm 16 MB/s | 1.2 MB 00:00
(22/64): bind-license-9.16.23-5.el9_1.noarch.rpm 403 kB/s | 13 kB 00:00
(23/64): bind-utils-9.16.23-5.el9_1.x86_64.rpm 3.8 MB/s | 224 kB 00:00
(24/64): checkpolicy-3.4-1.el9.x86_64.rpm 6.5 MB/s | 355 kB 00:00
(25/64): smartmontools-7.2-6.el9.x86_64.rpm 560 kB/s | 578 kB 00:01
(26/64): python3-setuptools-53.0.0-10.el9.noarch.rpm 482 kB/s | 1.3 MB 00:02
(27/64): fstrm-0.6.1-3.el9.x86_64.rpm 17 kB/s | 28 kB 00:01
(28/64): glibc-devel-2.34-40.0.1.el9.x86_64.rpm 40 kB/s | 62 kB 00:01
(29/64): glibc-headers-2.34-40.0.1.el9.x86_64.rpm 428 kB/s | 910 kB 00:02
(30/64): kernel-headers-5.14.0-162.6.1.el9_1.x86_64.rpm 1.6 MB/s | 4.2 MB 00:02
(31/64): libICE-1.0.10-8.el9.x86_64.rpm 40 kB/s | 71 kB 00:01
(32/64): ksh-1.0.0~beta.1-2.0.1.el9.x86_64.rpm 321 kB/s | 891 kB 00:02
(33/64): libX11-common-1.7.0-7.el9.noarch.rpm 10 MB/s | 350 kB 00:00
(34/64): libSM-1.2.3-10.el9.x86_64.rpm 30 kB/s | 42 kB 00:01
(35/64): libXau-1.0.9-8.el9.x86_64.rpm 1.6 MB/s | 36 kB 00:00
(36/64): libXcomposite-0.4.5-7.el9.x86_64.rpm 1.3 MB/s | 29 kB 00:00
(37/64): libXext-1.3.4-8.el9.x86_64.rpm 1.8 MB/s | 40 kB 00:00
(38/64): libX11-1.7.0-7.el9.x86_64.rpm 374 kB/s | 648 kB 00:01
(39/64): libXinerama-1.1.4-10.el9.x86_64.rpm 703 kB/s | 15 kB 00:00
(40/64): libX11-xcb-1.7.0-7.el9.x86_64.rpm 6.8 kB/s | 12 kB 00:01
(41/64): libXrandr-1.5.2-8.el9.x86_64.rpm 1.2 MB/s | 28 kB 00:00
(42/64): libXi-1.7.10-8.el9.x86_64.rpm 29 kB/s | 40 kB 00:01
(43/64): libXmu-1.1.3-8.el9.x86_64.rpm 56 kB/s | 79 kB 00:01
(44/64): libXrender-0.9.10-16.el9.x86_64.rpm 17 kB/s | 28 kB 00:01
(45/64): libXt-1.2.0-6.el9.x86_64.rpm 112 kB/s | 180 kB 00:01
(46/64): libXtst-1.2.3-16.el9.x86_64.rpm 15 kB/s | 21 kB 00:01
(47/64): libXxf86dga-1.1.5-8.el9.x86_64.rpm 15 kB/s | 21 kB 00:01
(48/64): libXv-1.0.11-16.el9.x86_64.rpm 11 kB/s | 19 kB 00:01
(49/64): libXxf86vm-1.1.4-18.el9.x86_64.rpm 13 kB/s | 19 kB 00:01
(50/64): libdmx-1.1.4-12.el9.x86_64.rpm 17 kB/s | 17 kB 00:01
(51/64): libmaxminddb-1.5.2-3.el9.x86_64.rpm 28 kB/s | 34 kB 00:01
(52/64): libuv-1.42.0-1.el9.x86_64.rpm 82 kB/s | 158 kB 00:01
(53/64): libxcb-1.13.1-9.el9.x86_64.rpm 144 kB/s | 251 kB 00:01
(54/64): libxcrypt-devel-4.4.18-3.el9.x86_64.rpm 26 kB/s | 42 kB 00:01
(55/64): lm_sensors-libs-3.6.0-10.el9.x86_64.rpm 42 kB/s | 42 kB 00:00
(56/64): policycoreutils-python-utils-3.4-4.el9.noarch.rpm 4.7 MB/s | 111 kB 00:00
(57/64): python3-audit-3.0.7-103.el9.x86_64.rpm 3.8 MB/s | 88 kB 00:00
(58/64): python3-libsemanage-3.4-2.el9.x86_64.rpm 1.4 MB/s | 85 kB 00:00
(59/64): python3-policycoreutils-3.4-4.el9.noarch.rpm 39 MB/s | 2.3 MB 00:00
(60/64): pcp-conf-5.3.7-7.el9.x86_64.rpm 28 kB/s | 38 kB 00:01
(61/64): pcp-libs-5.3.7-7.el9.x86_64.rpm 316 kB/s | 612 kB 00:01
(62/64): sysstat-12.5.4-3.0.1.el9.x86_64.rpm 246 kB/s | 539 kB 00:02
(63/64): xorg-x11-utils-7.5-40.el9.x86_64.rpm 62 kB/s | 123 kB 00:01
(64/64): xorg-x11-xauth-1.1-10.el9.x86_64.rpm 25 kB/s | 36 kB 00:01
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Total 1.1 MB/s | 25 MB 00:22
Running transaction check
Transaction check succeeded.
Running transaction test
Transaction test succeeded.
Running transaction
Preparing : 1/1
Installing : binutils-gold-2.35.2-24.0.1.el9.x86_64 1/65
Installing : binutils-2.35.2-24.0.1.el9.x86_64 2/65
Running scriptlet: binutils-2.35.2-24.0.1.el9.x86_64 2/65
Installing : libuv-1:1.42.0-1.el9.x86_64 3/65
Installing : libXau-1.0.9-8.el9.x86_64 4/65
Installing : libxcb-1.13.1-9.el9.x86_64 5/65
Installing : libICE-1.0.10-8.el9.x86_64 6/65
Installing : protobuf-c-1.3.3-12.el9.x86_64 7/65
Installing : libnfsidmap-1:2.5.4-15.el9.x86_64 8/65
Installing : libSM-1.2.3-10.el9.x86_64 9/65
Installing : python3-libsemanage-3.4-2.el9.x86_64 10/65
Installing : python3-audit-3.0.7-103.el9.x86_64 11/65
Installing : pcp-conf-5.3.7-7.el9.x86_64 12/65
Installing : pcp-libs-5.3.7-7.el9.x86_64 13/65
Installing : lm_sensors-libs-3.6.0-10.el9.x86_64 14/65
Installing : sysstat-12.5.4-3.0.1.el9.x86_64 15/65
Running scriptlet: sysstat-12.5.4-3.0.1.el9.x86_64 15/65
Created symlink /etc/systemd/system/multi-user.target.wants/sysstat.service → /usr/lib/systemd/system/sysstat.service.
Created symlink /etc/systemd/system/sysstat.service.wants/sysstat-collect.timer → /usr/lib/systemd/system/sysstat-collect.timer.
Created symlink /etc/systemd/system/sysstat.service.wants/sysstat-summary.timer → /usr/lib/systemd/system/sysstat-summary.timer.
Installing : libmaxminddb-1.5.2-3.el9.x86_64 16/65
Installing : libX11-xcb-1.7.0-7.el9.x86_64 17/65
Installing : libX11-common-1.7.0-7.el9.noarch 18/65
Installing : libX11-1.7.0-7.el9.x86_64 19/65
Installing : libXext-1.3.4-8.el9.x86_64 20/65
Installing : libXi-1.7.10-8.el9.x86_64 21/65
Installing : libXrender-0.9.10-16.el9.x86_64 22/65
Installing : libXrandr-1.5.2-8.el9.x86_64 23/65
Installing : libXtst-1.2.3-16.el9.x86_64 24/65
Installing : libXinerama-1.1.4-10.el9.x86_64 25/65
Installing : libXv-1.0.11-16.el9.x86_64 26/65
Installing : libXxf86dga-1.1.5-8.el9.x86_64 27/65
Installing : libXxf86vm-1.1.4-18.el9.x86_64 28/65
Installing : libdmx-1.1.4-12.el9.x86_64 29/65
Installing : libXcomposite-0.4.5-7.el9.x86_64 30/65
Installing : xorg-x11-utils-7.5-40.el9.x86_64 31/65
Installing : libXt-1.2.0-6.el9.x86_64 32/65
Installing : libXmu-1.1.3-8.el9.x86_64 33/65
Installing : xorg-x11-xauth-1:1.1-10.el9.x86_64 34/65
Installing : ksh-3:1.0.0~beta.1-2.0.1.el9.x86_64 35/65
Running scriptlet: ksh-3:1.0.0~beta.1-2.0.1.el9.x86_64 35/65
Installing : kernel-headers-5.14.0-162.6.1.el9_1.x86_64 36/65
Installing : glibc-headers-2.34-40.0.1.el9.x86_64 37/65
Installing : libxcrypt-devel-4.4.18-3.el9.x86_64 38/65
Installing : glibc-devel-2.34-40.0.1.el9.x86_64 39/65
Installing : fstrm-0.6.1-3.el9.x86_64 40/65
Installing : checkpolicy-3.4-1.el9.x86_64 41/65
Installing : bind-license-32:9.16.23-5.el9_1.noarch 42/65
Installing : bind-libs-32:9.16.23-5.el9_1.x86_64 43/65
Installing : bind-utils-32:9.16.23-5.el9_1.x86_64 44/65
Installing : unzip-6.0-56.0.1.el9.x86_64 45/65
Installing : smartmontools-1:7.2-6.el9.x86_64 46/65
Running scriptlet: smartmontools-1:7.2-6.el9.x86_64 46/65
Created symlink /etc/systemd/system/multi-user.target.wants/smartd.service → /usr/lib/systemd/system/smartd.service.
Running scriptlet: rpcbind-1.2.6-5.el9.x86_64 47/65
Installing : rpcbind-1.2.6-5.el9.x86_64 47/65
Running scriptlet: rpcbind-1.2.6-5.el9.x86_64 47/65
Created symlink /etc/systemd/system/multi-user.target.wants/rpcbind.service → /usr/lib/systemd/system/rpcbind.service.
Created symlink /etc/systemd/system/sockets.target.wants/rpcbind.socket → /usr/lib/systemd/system/rpcbind.socket.
Installing : quota-nls-1:4.06-6.el9.noarch 48/65
Installing : quota-1:4.06-6.el9.x86_64 49/65
Installing : python3-setuptools-53.0.0-10.el9.noarch 50/65
Installing : python3-setools-4.4.0-5.el9.x86_64 51/65
Installing : python3-policycoreutils-3.4-4.el9.noarch 52/65
Installing : policycoreutils-python-utils-3.4-4.el9.noarch 53/65
Installing : python3-pyyaml-5.4.1-6.0.1.el9.x86_64 54/65
Installing : net-tools-2.0-0.62.20160912git.el9.x86_64 55/65
Running scriptlet: net-tools-2.0-0.62.20160912git.el9.x86_64 55/65
Installing : libnsl-2.34-40.0.1.el9.x86_64 56/65
Installing : libev-4.33-5.el9.x86_64 57/65
Installing : libverto-libev-0.3.2-3.el9.x86_64 58/65
Installing : gssproxy-0.8.4-4.el9.x86_64 59/65
Running scriptlet: gssproxy-0.8.4-4.el9.x86_64 59/65
Installing : keyutils-1.6.1-4.el9.x86_64 60/65
Running scriptlet: nfs-utils-1:2.5.4-15.el9.x86_64 61/65
Installing : nfs-utils-1:2.5.4-15.el9.x86_64 61/65
Running scriptlet: nfs-utils-1:2.5.4-15.el9.x86_64 61/65
Installing : initscripts-10.11.5-1.el9.x86_64 62/65
Running scriptlet: initscripts-10.11.5-1.el9.x86_64 62/65
Created symlink /etc/systemd/system/sysinit.target.wants/import-state.service → /usr/lib/systemd/system/import-state.service.
Created symlink /etc/systemd/system/sysinit.target.wants/loadmodules.service → /usr/lib/systemd/system/loadmodules.service.
Installing : bc-1.07.1-14.el9.x86_64 63/65
Installing : oracle-database-preinstall-21c-1.0-1.el8.x86_64 64/65
Installing : sssd-nfs-idmap-2.7.3-4.0.1.el9_1.1.x86_64 65/65
Running scriptlet: oracle-database-preinstall-21c-1.0-1.el8.x86_64 65/65
Running scriptlet: sssd-nfs-idmap-2.7.3-4.0.1.el9_1.1.x86_64 65/65
Verifying : bc-1.07.1-14.el9.x86_64 1/65
Verifying : binutils-2.35.2-24.0.1.el9.x86_64 2/65
Verifying : binutils-gold-2.35.2-24.0.1.el9.x86_64 3/65
Verifying : gssproxy-0.8.4-4.el9.x86_64 4/65
Verifying : initscripts-10.11.5-1.el9.x86_64 5/65
Verifying : keyutils-1.6.1-4.el9.x86_64 6/65
Verifying : libev-4.33-5.el9.x86_64 7/65
Verifying : libnfsidmap-1:2.5.4-15.el9.x86_64 8/65
Verifying : libnsl-2.34-40.0.1.el9.x86_64 9/65
Verifying : libverto-libev-0.3.2-3.el9.x86_64 10/65
Verifying : net-tools-2.0-0.62.20160912git.el9.x86_64 11/65
Verifying : nfs-utils-1:2.5.4-15.el9.x86_64 12/65
Verifying : protobuf-c-1.3.3-12.el9.x86_64 13/65
Verifying : python3-pyyaml-5.4.1-6.0.1.el9.x86_64 14/65
Verifying : python3-setools-4.4.0-5.el9.x86_64 15/65
Verifying : python3-setuptools-53.0.0-10.el9.noarch 16/65
Verifying : quota-1:4.06-6.el9.x86_64 17/65
Verifying : quota-nls-1:4.06-6.el9.noarch 18/65
Verifying : rpcbind-1.2.6-5.el9.x86_64 19/65
Verifying : smartmontools-1:7.2-6.el9.x86_64 20/65
Verifying : sssd-nfs-idmap-2.7.3-4.0.1.el9_1.1.x86_64 21/65
Verifying : unzip-6.0-56.0.1.el9.x86_64 22/65
Verifying : bind-libs-32:9.16.23-5.el9_1.x86_64 23/65
Verifying : bind-license-32:9.16.23-5.el9_1.noarch 24/65
Verifying : bind-utils-32:9.16.23-5.el9_1.x86_64 25/65
Verifying : checkpolicy-3.4-1.el9.x86_64 26/65
Verifying : fstrm-0.6.1-3.el9.x86_64 27/65
Verifying : glibc-devel-2.34-40.0.1.el9.x86_64 28/65
Verifying : glibc-headers-2.34-40.0.1.el9.x86_64 29/65
Verifying : kernel-headers-5.14.0-162.6.1.el9_1.x86_64 30/65
Verifying : ksh-3:1.0.0~beta.1-2.0.1.el9.x86_64 31/65
Verifying : libICE-1.0.10-8.el9.x86_64 32/65
Verifying : libSM-1.2.3-10.el9.x86_64 33/65
Verifying : libX11-1.7.0-7.el9.x86_64 34/65
Verifying : libX11-common-1.7.0-7.el9.noarch 35/65
Verifying : libX11-xcb-1.7.0-7.el9.x86_64 36/65
Verifying : libXau-1.0.9-8.el9.x86_64 37/65
Verifying : libXcomposite-0.4.5-7.el9.x86_64 38/65
Verifying : libXext-1.3.4-8.el9.x86_64 39/65
Verifying : libXi-1.7.10-8.el9.x86_64 40/65
Verifying : libXinerama-1.1.4-10.el9.x86_64 41/65
Verifying : libXmu-1.1.3-8.el9.x86_64 42/65
Verifying : libXrandr-1.5.2-8.el9.x86_64 43/65
Verifying : libXrender-0.9.10-16.el9.x86_64 44/65
Verifying : libXt-1.2.0-6.el9.x86_64 45/65
Verifying : libXtst-1.2.3-16.el9.x86_64 46/65
Verifying : libXv-1.0.11-16.el9.x86_64 47/65
Verifying : libXxf86dga-1.1.5-8.el9.x86_64 48/65
Verifying : libXxf86vm-1.1.4-18.el9.x86_64 49/65
Verifying : libdmx-1.1.4-12.el9.x86_64 50/65
Verifying : libmaxminddb-1.5.2-3.el9.x86_64 51/65
Verifying : libuv-1:1.42.0-1.el9.x86_64 52/65
Verifying : libxcb-1.13.1-9.el9.x86_64 53/65
Verifying : libxcrypt-devel-4.4.18-3.el9.x86_64 54/65
Verifying : lm_sensors-libs-3.6.0-10.el9.x86_64 55/65
Verifying : pcp-conf-5.3.7-7.el9.x86_64 56/65
Verifying : pcp-libs-5.3.7-7.el9.x86_64 57/65
Verifying : policycoreutils-python-utils-3.4-4.el9.noarch 58/65
Verifying : python3-audit-3.0.7-103.el9.x86_64 59/65
Verifying : python3-libsemanage-3.4-2.el9.x86_64 60/65
Verifying : python3-policycoreutils-3.4-4.el9.noarch 61/65
Verifying : sysstat-12.5.4-3.0.1.el9.x86_64 62/65
Verifying : xorg-x11-utils-7.5-40.el9.x86_64 63/65
Verifying : xorg-x11-xauth-1:1.1-10.el9.x86_64 64/65
Verifying : oracle-database-preinstall-21c-1.0-1.el8.x86_64 65/65
Installed:
bc-1.07.1-14.el9.x86_64 bind-libs-32:9.16.23-5.el9_1.x86_64 bind-license-32:9.16.23-5.el9_1.noarch bind-utils-32:9.16.23-5.el9_1.x86_64 binutils-2.35.2-24.0.1.el9.x86_64
binutils-gold-2.35.2-24.0.1.el9.x86_64 checkpolicy-3.4-1.el9.x86_64 fstrm-0.6.1-3.el9.x86_64 glibc-devel-2.34-40.0.1.el9.x86_64 glibc-headers-2.34-40.0.1.el9.x86_64
gssproxy-0.8.4-4.el9.x86_64 initscripts-10.11.5-1.el9.x86_64 kernel-headers-5.14.0-162.6.1.el9_1.x86_64 keyutils-1.6.1-4.el9.x86_64 ksh-3:1.0.0~beta.1-2.0.1.el9.x86_64
libICE-1.0.10-8.el9.x86_64 libSM-1.2.3-10.el9.x86_64 libX11-1.7.0-7.el9.x86_64 libX11-common-1.7.0-7.el9.noarch libX11-xcb-1.7.0-7.el9.x86_64
libXau-1.0.9-8.el9.x86_64 libXcomposite-0.4.5-7.el9.x86_64 libXext-1.3.4-8.el9.x86_64 libXi-1.7.10-8.el9.x86_64 libXinerama-1.1.4-10.el9.x86_64
libXmu-1.1.3-8.el9.x86_64 libXrandr-1.5.2-8.el9.x86_64 libXrender-0.9.10-16.el9.x86_64 libXt-1.2.0-6.el9.x86_64 libXtst-1.2.3-16.el9.x86_64
libXv-1.0.11-16.el9.x86_64 libXxf86dga-1.1.5-8.el9.x86_64 libXxf86vm-1.1.4-18.el9.x86_64 libdmx-1.1.4-12.el9.x86_64 libev-4.33-5.el9.x86_64
libmaxminddb-1.5.2-3.el9.x86_64 libnfsidmap-1:2.5.4-15.el9.x86_64 libnsl-2.34-40.0.1.el9.x86_64 libuv-1:1.42.0-1.el9.x86_64 libverto-libev-0.3.2-3.el9.x86_64
libxcb-1.13.1-9.el9.x86_64 libxcrypt-devel-4.4.18-3.el9.x86_64 lm_sensors-libs-3.6.0-10.el9.x86_64 net-tools-2.0-0.62.20160912git.el9.x86_64 nfs-utils-1:2.5.4-15.el9.x86_64
oracle-database-preinstall-21c-1.0-1.el8.x86_64 pcp-conf-5.3.7-7.el9.x86_64 pcp-libs-5.3.7-7.el9.x86_64 policycoreutils-python-utils-3.4-4.el9.noarch protobuf-c-1.3.3-12.el9.x86_64
python3-audit-3.0.7-103.el9.x86_64 python3-libsemanage-3.4-2.el9.x86_64 python3-policycoreutils-3.4-4.el9.noarch python3-pyyaml-5.4.1-6.0.1.el9.x86_64 python3-setools-4.4.0-5.el9.x86_64
python3-setuptools-53.0.0-10.el9.noarch quota-1:4.06-6.el9.x86_64 quota-nls-1:4.06-6.el9.noarch rpcbind-1.2.6-5.el9.x86_64 smartmontools-1:7.2-6.el9.x86_64
sssd-nfs-idmap-2.7.3-4.0.1.el9_1.1.x86_64 sysstat-12.5.4-3.0.1.el9.x86_64 unzip-6.0-56.0.1.el9.x86_64 xorg-x11-utils-7.5-40.el9.x86_64 xorg-x11-xauth-1:1.1-10.el9.x86_64
Complete!
Install Database XE RPM
dnf -y localinstall oracle-database-xe-21c-1.0-1.ol8.x86_64.rpm
Last metadata expiration check: 3:43:34 ago on Thu 22 Dec 2022 03:25:41 AM EST.
Dependencies resolved.
=============================================================================================================================================================================================================================================
Package Architecture Version Repository Size
=============================================================================================================================================================================================================================================
Installing:
oracle-database-xe-21c x86_64 1.0-1 @commandline 2.2 G
Transaction Summary
=============================================================================================================================================================================================================================================
Install 1 Package
Total size: 2.2 G
Installed size: 5.8 G
Downloading Packages:
Running transaction check
Transaction check succeeded.
Running transaction test
Transaction test succeeded.
Running transaction
Preparing : 1/1
Running scriptlet: oracle-database-xe-21c-1.0-1.x86_64 1/1
Installing : oracle-database-xe-21c-1.0-1.x86_64 1/1
Running scriptlet: oracle-database-xe-21c-1.0-1.x86_64 1/1
[INFO] Executing post installation scripts...
[INFO] Oracle home installed successfully and ready to be configured.
To configure Oracle Database XE, optionally modify the parameters in '/etc/sysconfig/oracle-xe-21c.conf' and then execute '/etc/init.d/oracle-xe-21c configure' as root.
Verifying : oracle-database-xe-21c-1.0-1.x86_64 1/1
Installed:
oracle-database-xe-21c-1.0-1.x86_64
Complete!
This Completes the Required RPM and Database Installation
Configuring the Database
# /etc/init.d/oracle-xe-21c configure
Oracle Net Listener configured.
Specify a password to be used for database accounts. Oracle recommends that the password entered should be at least 8 characters in length, contain at least 1 uppercase character, 1 lower case character and 1 digit [0-9]. Note that the same password will be used for SYS, SYSTEM and PDBADMIN accounts:
Confirm the password:
Configuring Oracle Listener.
Listener configuration succeeded.
Configuring Oracle Database XE.
Enter SYS user password:
************
Enter SYSTEM user password:
**************
Enter PDBADMIN User Password:
**************
[WARNING] [INS-08109] Unexpected error occurred while validating inputs at state 'DBCreationOptions'.
CAUSE: No additional information available.
ACTION: Contact Oracle Support Services or refer to the software manual.
SUMMARY:
- java.lang.NullPointerException
Database configuration failed. Check logs under '/opt/oracle/cfgtoollogs/dbca'.
Set the CV_ASSUME_DISTID variable as its coming as NULL, to proceed further.
export CV_ASSUME_DISTID=OEL8.4
# /etc/init.d/oracle-xe-21c configure
Oracle Net Listener configured.
Specify a password to be used for database accounts. Oracle recommends that the password entered should be at least 8 characters in length, contain at least 1 uppercase character, 1 lower case character and 1 digit [0-9]. Note that the same password will be used for SYS, SYSTEM and PDBADMIN accounts:
Confirm the password:
Configuring Oracle Listener.
Listener configuration succeeded.
Configuring Oracle Database XE.
Enter SYS user password:
*************
Enter SYSTEM user password:
*************
Enter PDBADMIN User Password:
************
Prepare for db operation
7% complete
Copying database files
29% complete
Creating and starting Oracle instance
30% complete
33% complete
37% complete
40% complete
43% complete
Completing Database Creation
47% complete
50% complete
Creating Pluggable Databases
54% complete
71% complete
Executing Post Configuration Actions
93% complete
Running Custom Scripts
100% complete
Database creation complete. For details check the logfiles at:
/opt/oracle/cfgtoollogs/dbca/XE.
Database Information:
Global Database Name:XE
System Identifier(SID):XE
Look at the log file "/opt/oracle/cfgtoollogs/dbca/XE/XE.log" for further details.
Connect to Oracle Database using one of the connect strings:
Pluggable database: masked_FQDN:1523/XEPDB1
Multitenant container database: masked_FQDN:1523
Use https://localhost:5500/em to access Oracle Enterprise Manager for Oracle Database XE
This Completes the Oracle Database 21c Express Edition Installation on Oracle Linux 9
$ sqlplus /nolog
SQL*Plus: Release 21.0.0.0.0 - Production on Thu Dec 22 08:03:44 2022
Version 21.3.0.0.0
Copyright (c) 1982, 2021, Oracle. All rights reserved.
SQL> exit
Disconnected from Oracle Database 21c Express Edition Release 21.0.0.0.0 - Production
Version 21.3.0.0.0
Hope this is useful. , Have a good day.
Posted in oracle, Oracle Administration, Oracle Database 21c, Oracle Server Administration, Oracle XE | Tagged: 21c, Installation, Linux, linux 9, ol9, RPM, xe, YUM | 1 Comment »
Sample Script to Publish a blog Post #Python
Posted by Sriram Sanka on March 29, 2023
Sample Script to Publish a blog Post Using Python
This post is Auto published using Python script attached below. #Python
import json
from wordpress_xmlrpc import Client, WordPressPost
from wordpress_xmlrpc.methods.posts import NewPost
import getpass
password = getpass.getpass(prompt='Password: ', stream=None) //Blog Login Password
def auto_blog_post(blog_content,blog_excerpt,blog_status):
id = 'sriramoracle' //User Name
url = 'https://sriramoracle.wordpress.com/xmlrpc.php'
wp = Client(url, id, password)
post = WordPressPost()
post.post_status = blog_status
post.title = blog_excerpt
post.content = blog_content
post.excerpt = blog_excerpt
post.terms_names = {
"post_tag": ['Python'],
"category": ['Python']
}
wp.call(NewPost(post))
auto_blog_post('Sample Script to Publish a blog Post Using Python ','Sample Script to Publish a blog Post ' ,'publish') //publish will publish the Post, Draft is the default mode.
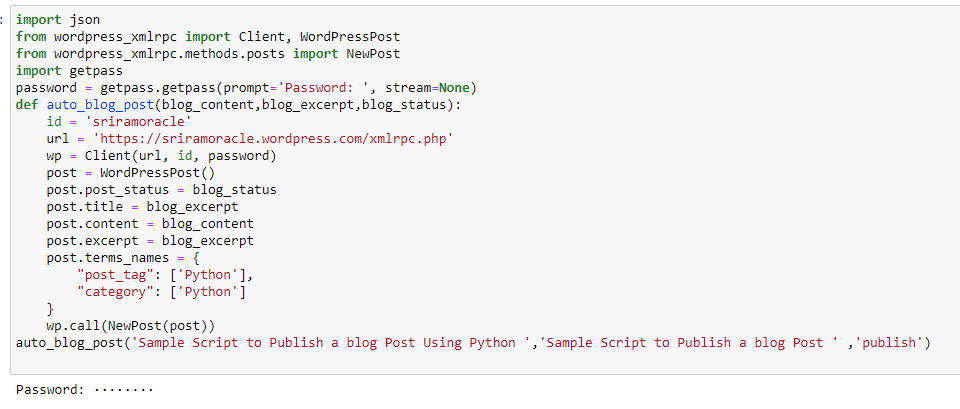
Posted in Python | Tagged: Python | Leave a Comment »